Historical Volatility Formula in Python:A Guide to Using Python for Historical Volatility Calculations
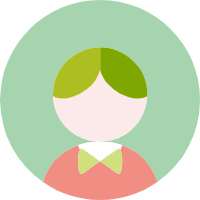
Historical volatility, also known as past volatility, is the level of price volatility for a security or portfolio over a specific time period, typically one year. It is an important metric for investors and financial analysts, as it provides a measure of the price fluctuations of a security or market over time. In this article, we will explore the historical volatility formula in Python and how to use the programming language for historical volatility calculations. We will also provide a step-by-step guide on how to implement the formula and its various applications.
Historical Volatility Formula
The historical volatility formula can be calculated using the following equation:
ΔR = sqrt(Σ(ΔP_i)^2 / Σ(ΔT_i)^2)
Where:
ΔR = Change in Returns
ΔP_i = Changes in Prices for each time period
ΔT_i = Time periods for each change in price
Step-by-Step Guide to Calculating Historical Volatility in Python
1. Import Required Libraries
In order to calculate historical volatility in Python, we need to import the following libraries:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
```
2. Load Data
Load the required data (stock prices or index prices) into a pandas DataFrame. The data should include the close prices for each trading day, as well as the date and exchange the security trades on.
```python
data = pd.read_csv('stock_prices.csv', index_col='Date', parse_dates=True)
```
3. Calculate Returns
Calculate the annualized returns for each trading day by dividing the final close price by the opening price for that day.
```python
annual_returns = (data['Close'] / data['Open']).pct_change() * 252
```
4. Calculate Volatility
Calculate the annualized historical volatility using the formula provided above.
```python
historical_volatility = np.sqrt(np.sum((annual_returns.diff() ** 2) / np.sum((annual_returns.diff().abs() ** 2) / (annual_returns.diff().shift(1).abs() ** 2)))
```
5. Plot Volatility
Plot the historical volatility against the dates for which it was calculated.
```python
plt.plot(data.index, historical_volatility)
plt.xlabel('Date')
plt.ylabel('Historical Volatility')
plt.title('Historical Volatility vs Date')
plt.show()
```
Applications of Historical Volatility
Historical volatility is a valuable tool for investors and financial analysts, as it provides a measure of the price fluctuations of a security or market over time. Some applications of historical volatility include:
- Portfolio optimization: Investors can use historical volatility to understand the risk associated with their portfolio and make better investment decisions.
- Option pricing: Historical volatility is a key input in the valuation of options, such as call and put options.
- Risk management: Financial institutions can use historical volatility to gauge the risk associated with their investments and make better risk management decisions.
In this article, we have explored the historical volatility formula in Python and provided a step-by-step guide on how to implement the formula and its various applications. Historical volatility is an important metric for investors and financial analysts, as it provides a measure of the price fluctuations of a security or market over time. By understanding how to calculate historical volatility in Python, investors and financial analysts can make more informed decisions and better understand the risks associated with their investments.